Python comes with a built-in try… except syntax with which you can handle errors and stop them from interrupting the running of your program.
How To Fix “Python try except print error fix”
Here are several solutions to tackle this error and get your programs running smoothly and some solutions suggested by Stackoverflow users to help you resolve this error.
1. Directly Printing the Exception
- You can use the following syntax in Python 3.X and Python 2.6 and later:
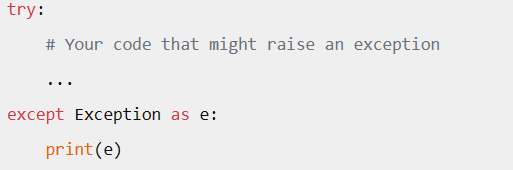
- Use this for Python 2.5 and older:
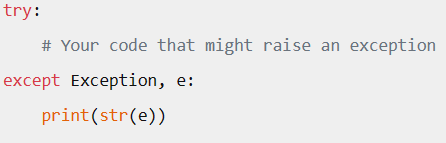
2. Understanding the Try-Except Block
At its core, the try-except
block serves as a mechanism for handling exceptions, or errors, that occur during program execution. The structure is simple:
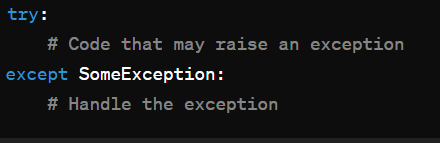
Within the try
block, you place the code that may potentially raise an exception. If an exception occurs, Python searches for a matching except
block. If found, the code within the corresponding except
block is executed, allowing the program to handle the error gracefully.
3. Handling Specific Exceptions
Python offers a variety of built-in exception types to cover a wide range of potential errors. By specifying the type of exception in the except
block, you can tailor your error handling to address specific scenarios. For example:
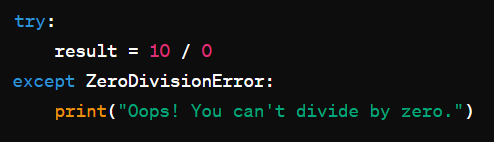
In this example, the ZeroDivisionError
exception is caught, and a user-friendly error message is displayed, preventing the program from crashing due to division by zero.
4. Handling Multiple Exception
You may encounter multiple exceptions within a single block in more complex scenarios. Python allows you to handle each exception type separately by including multiple except blocks. For instance:
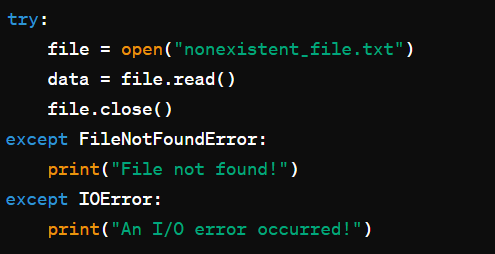
Here, the code attempts to open a file and read its contents. If the file does not exist (FileNotFoundError
) or an I/O error occurs (IOError
), appropriate error messages are printed, preventing the program from crashing.
5. Using the Traceback Module
- If you need more detailed information, consider using the
traceback
module. It provides methods for formatting and printing exceptions and their tracebacks. - For example, to print the full traceback without halting the program:
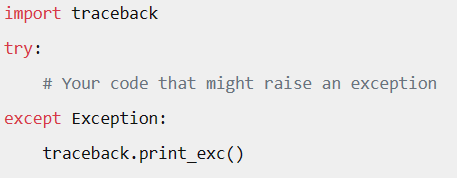